A list is a sequence of several variables, grouped together under a single name. Instead of writing a program with many variables x0
, x1
, x2
, … you can define a single variable x
and access its members x[0]
, x[1]
, x[2]
, etc. More importantly, you can put other expressions and variables inside the square brackets, like x[i]
and x[i+1]
. This allows us to deal with arbitrarily large data sets using just a single small piece of code.
One way to create a list is by enclosing several values, separated with commas, between square brackets:
myList = ["the first value in the list", 1999, 4.5]This creates a list called
myList
of length 3. Each element of the list gets a number called its index: the initial element has index 0, the next element has index 1, and so forth. The individual variables comprising the list have the names
«listName»[«indexNumber»]
So in this example, myList[0]
is a variable whose value is the string "the first value in the list"
and print(myList[2])
would print 4.5
. You can also change the values of items in the list, and print out entire lists:
As you can see, numbers[0]
is treated as if it were itself a variable: it has a value and can be changed.
Next, try to predict the final state of the visualized example below, then compare it with actually running the code.
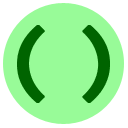
stuff = [2, 25, 80, 12]
stuff[stuff[0]] = stuff[3]
print(stuff)
stuff[3]
on the right side is 12
. For the left side, stuff[0]
is 2
, so stuff[stuff[0]]
refers to the variable stuff[2]
. The value of this variable is updated (from 80
) to 12
.![]() | What Python calls a list would be called an array in most other programming languages. Python also has something different & more advanced called arrays. |
A Common Error
If you try to ask Python for an index that doesn't exist, it gives you an error:
In the above example, because myList
has length 4 and the first index is 0, the maximum possible index is 3. Asking for an index of 4, 5, or anything larger gives this kind of error.
Common Useful Operations
The Length of a List: len(«list»)
To determine the number of items in a list, call the function len()
on that list. Look at how range
is used in the following example.
It is common to use len
to write code that can work on lists of any length, like in the example above, and in the next exercise.
Are Lists Like Strings?
At this point, you might have noticed that the operations on lists are a lot like strings: both of them can be passed to the len()
function to get their lengths, and both of them use X[«index»]
to extract individual items. Lists and strings are indeed related: they are both "sequence types" in Python. The one major difference is that individual characters in strings cannot be changed.
For this reason lists are called a mutable type and strings are immutable; you will see a little more information about this in lesson 17.
Concatenation and Creation
From the lesson about the str
type, you may remember that it is possible to use +
to merge (concatenate) two strings together. You can do the same thing with lists:
Similarly, you can use the multiplication symbol *
to extend a list by repetition. This is useful for creating a new list of a desired length.
To solve the next exercise, use one of the operators we just introduced, and a for
loop.
End of the Line: Negative Indices
To get the last item of a list, use
«listName»[-1]
More generally, L[-k]
returns the k
th item from the end of the list; Python handles this internally by translating it to L[len(L)-k]
. This shortcut notation works for strings too!
max
and sum
The function max
which we saw before can also be applied to a list of numbers: it returns the largest number in the list. Likewise, the function sum(L)
returns the sum of the elements in list L
.
Looping through lists
It is very common (like in the previous exercise) to loop through every value in a list. Python allows a shortcut to perform this type of an operation, usually called a "for all" loop or a "for each" loop. Specifically, when L is a list, this code
for x in L: «loop body block»does the following: first
x
is set to the first value in L
and the body is executed; then x
is set to the second value in L
and the body is executed; this is continued for all items in L
.
Here is a visualized example of printing out the elements in a list:
"For all" loops work for strings too: try for char in "hello"
.
Well done! you can proceed to the next lesson, or try some bonus exercises below.
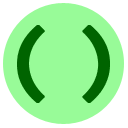
x
which will cause mystery(x)
to run forever?
def mystery(x):
a = [0, 4, 0, 3, 2]
while x > 0:
x = a[x]
return "Done"